Using environment variables with Spring Boot
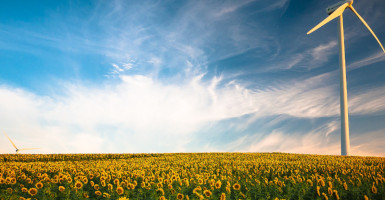
This post will cover how you can use OS environment variables to pass configuration values to a Spring Boot application.
How to write a good commit message
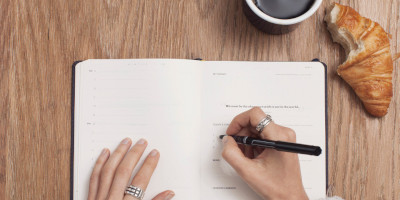
Version control systems play a vital role in tracking the history of changes in a codebase. Knowing how to write good commit messages is an essential skill if you wish to have your project’s history well documented.
takeWhile and dropWhile in Java 9 Streams
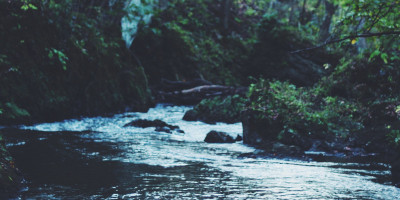
Arguably one of the most noteworthy new features introduced in Java 8 was the Streams API. Java 9 offers two new stream operations—takeWhile and dropWhile.
To field inject, or not to field inject in Spring?
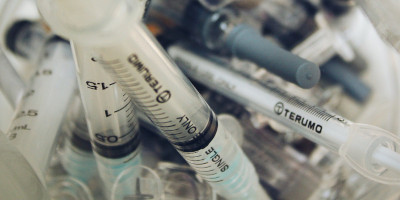
Nearly all Spring projects that I have worked with make heavy use of field injection. This must be a popular approach because it is concise and reads well. But have you ever considered the downsides of field injection?
Java’s While and Do-While Loops in Five Minutes
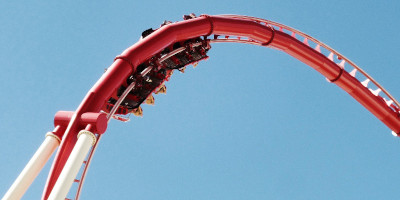
A while loop is a control flow statement that runs a piece of code multiple times. It consists of a loop condition and body. Java also has a do while loop.
Java’s Ternary Operator in Three Minutes
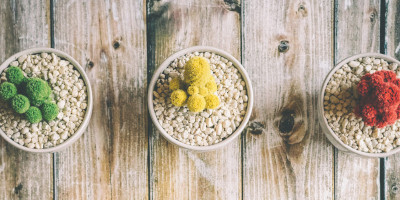
The ternary operator is a form of syntactic sugar for if-then-else statements. It is also known as the conditional operator, which is perhaps a more meaningful name because it evaluates conditions like if does.
Java’s If Statement in Five Minutes
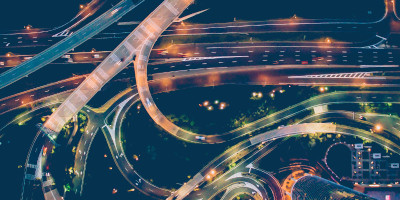
The if statement is the most basic conditional statement. It checks a condition, which is any boolean expression, and runs a block of code if it is true.
Unit testing classes that depend on time
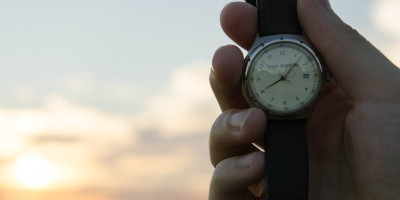
Dependency inversion principle states that we should depend upon abstractions. Taking that into account, time should also be considered as a dependency.
Type-safe configuration in Spring Boot
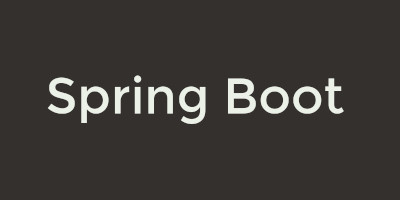
Spring Boot provides a type-safe way to handle configuration by allowing you to create a bean and populating it with property values from your configuration file.